01. DTO
1) ๐MyComment1.java - ํ ์ด๋ธ์ "์ปฌ๋ผ๋ช "๊ณผ "ํ๋๋ช "์ด ๊ฐ์
package xyz.itwill.dto;
import java.io.Serializable;
/*
MYCOMMENT ํ
์ด๋ธ : ๊ฒ์๊ธ์ ์ ์ฅํ๊ธฐ ์ํ ํ
์ด๋ธ
create table mycomment(comment_no number primary key, comment_id varchar2(50)
, comment_content varchar2(100), comment_date date );
//MYCOMMENT_SEQ ์ํ์ค : MYCOMMENT ํ
์ด๋ธ์ COMMENT_NO ์ปฌ๋ผ์ ์ ์ฅ๋ ์๋ ์ฆ๊ฐ๊ฐ์ ์ ๊ณตํ๊ธฐ ์ํ ์ํ์ค
create sequence mycomment_seq;
์ด๋ฆ ๋? ์ ํ
--------------- -------- -------------
COMMENT_NO NOT NULL NUMBER - ๊ฒ์๊ธ ๋ฒํธ
COMMENT_ID VARCHAR2(50) - ๊ฒ์๊ธ ์์ฑ์(์์ด๋)
COMMENT_CONTENT VARCHAR2(100) - ๊ฒ์๊ธ ๋ด์ฉ
COMMENT_DATE DATE - ๊ฒ์๊ธ ์์ฑ์ผ
*/
//ํ
์ด๋ธ์ ์ปฌ๋ผ๋ช
์ ๊ฐ์ ์ด๋ฆ์ ํ๋๋ฅผ ์ ์ธํ์ฌ ํด๋์ค ์์ฑ
//XML ๊ธฐ๋ฐ์ ๋งคํผ ํ์ผ์์ cache ์๋ฆฌ๋จผํธ๋ฅผ ์ฌ์ฉํ ๊ฒฝ์ฐ SELECT ๋ช
๋ น์ ๋ํ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ๊ณต๋
//๊ฐ์ฒด์ ํด๋์ค๋ ๋ฐ๋์ ๊ฐ์ฒด ์ง๋ ฌํ ํด๋์ค๋ก ์ ์ธ
//=> ๊ฐ์ฒด ์ง๋ ฌํ ํด๋์ค๋ Serializable ์ธํฐํ์ด์ค๋ฅผ ์์๋ฐ์ ์์ฑ
//=> ๊ฐ์ฒด ์ง๋ ฌํ ํด๋์ค๋ serialVersionUID์ด๋ฆ์ static final ํ๋ ์ ์ธํ๋ ๊ฒ์ ๊ถ์ฅ
public class MyComment1 implements Serializable{
private static final long serialVersionUID = 1L;
private int commentNo;
private String commentId;
private String commentContent;
private String commentDate;
public MyComment1() { }
public int getCommentNo() {return commentNo;}
public void setCommentNo(int commentNo) {this.commentNo = commentNo;}
public String getCommentId() {return commentId;}
public void setCommentId(String commentId) {this.commentId = commentId;}
public String getCommentContent() {return commentContent;}
public void setCommentContent(String commentContent) {this.commentContent = commentContent;}
public String getCommentDate() {return commentDate;}
public void setCommentDate(String commentDate) {this.commentDate = commentDate;}
}
2) ๐MyComment2.java - ํ ์ด๋ธ์ "์ปฌ๋ผ๋ช "๊ณผ "ํ๋๋ช "์ด ๋ค๋ฆ
package xyz.itwill.dto;
//ํ
์ด๋ธ์ ์ปฌ๋ผ๋ช
๊ณผ ๋ค๋ฅธ ์ด๋ฆ์ ํ๋๋ฅผ ์ ์ธํ์ฌ ํด๋์ค ์์ฑ
public class MyComment2 {
private int no;
private String id;
private String content;
private String date;
public MyComment2() { }
public MyComment2(int no, String id) {
super();
this.no = no;
this.id = id;
}
public MyComment2(int no, String id, String content, String date) {
super();
this.no = no;
this.id = id;
this.content = content;
this.date = date;
}
public int getNo() {return no;}
public void setNo(int no) {this.no = no;}
public String getId() {return id;}
public void setId(String id) {this.id = id;}
public String getContent() {return content;}
public void setContent(String content) {this.content = content;}
public String getDate() {return date;}
public void setDate(String date) {this.date = date;}
}
3) ๐MyComment3.java - ํ ์ด๋ธ์ "์ปฌ๋ผ๋ช "๊ณผ "ํ๋๋ช "์ด ๋ค๋ฆ
package xyz.itwill.dto;
//MYCOMMENT ํ
์ด๋ธ๊ณผ MYUSER ํ
์ด๋ธ์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
// => 1:1 ๊ด๊ณ์ ์กฐ์ธ ๊ฒ์ ๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
// => 1:1 ๊ด๊ณ์ ์กฐ์ธ : ํ
์ด๋ธ๊ณผ ํ
์ด๋ธ์ด ๋ง๋ ํ๋์ ํ์ผ๋ก ๊ฒ์๋จ (๋ฌด์กฐ๊ฑด inner ์กฐ์ธ ์ด์ฉ)
public class MyComment3 {
private int no;
private String id;
private String name; //MYCOMMENT ํ
์ด๋ธ์ด๋ MYUSER ํ
์ด๋ธ ์ค ์ด๋ค ์ปฌ๋ผ๊ฐ์ ์ ์ฅํด๋ ์๊ด์์
private String content;
private String date;
public MyComment3() { }
public int getNo() {return no;}
public void setNo(int no) {this.no = no;}
public String getId() {return id;}
public void setId(String id) {this.id = id;}
public String getName() {return name;}
public void setName(String name) {this.name = name;}
public String getContent() {return content;}
public void setContent(String content) {this.content = content;}
public String getDate() {return date;}
public void setDate(String date) {this.date = date;}
}
4) ๐MyCommentUser1.java - ํ๋์ ๊ฐ ์ ์ฅ (๋น๊ถ์ฅ) - 1:1 ๊ด๊ณ
package xyz.itwill.dto;
//MYCOMMENT ํ
์ด๋ธ๊ณผ MYUSER ํ
์ด๋ธ์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
//=> 1:1 ๊ด๊ณ์ ์กฐ์ธ ๊ฒ์ ๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
public class MyCommentUser1 {
//MYCOMMENT ํ
์ด๋ธ(๊ฒ์๊ธ์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
private int commentNo;
private String commentId;
private String commentContent;
private String commentDate;
//MYUSER ํ
์ด๋ธ(ํ์์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
private String userId;
private String userName;
public MyCommentUser1() { }
public int getCommentNo() {return commentNo;}
public void setCommentNo(int commentNo) {this.commentNo = commentNo;}
public String getCommentId() {return commentId;}
public void setCommentId(String commentId) {this.commentId = commentId;}
public String getCommentContent() {return commentContent;}
public void setCommentContent(String commentContent) {this.commentContent = commentContent;}
public String getCommentDate() {return commentDate;}
public void setCommentDate(String commentDate) {this.commentDate = commentDate;}
public String getUserId() {return userId;}
public void setUserId(String userId) {this.userId = userId;}
public String getUserName() {return userName;}
public void setUserName(String userName) {this.userName = userName;}
}
5) ๐ MyCommentUser2.java - ํ๋์ ๊ฐ์ฒด ์ ์ฅ (๊ถ์ฅ) - 1:1 ๊ด๊ณ
- ์์ฐ์ฑ ์ฆ๊ฐ ๋ฐ ์ ์ง๋ณด์์ ํจ์จ์ฑ ์ฆ๊ฐ
package xyz.itwill.dto;
//MYCOMMENT ํ
์ด๋ธ๊ณผ MYUSER ํ
์ด๋ธ์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
//=> 1:1 ๊ด๊ณ์ ์กฐ์ธ ๊ฒ์ ๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
//๊ธฐ์กด์ ์ ์ธ๋ ํด๋์ค(POZO : Plan Old Java Object)๋ฅผ ์ฌ์ฌ์ฉํ์ฌ ์๋ก์ด ํด๋์ค ์์ฑ
//=> ์์ฐ์ฑ ์ฆ๊ฐ ๋ฐ ์ ์ง๋ณด์์ ํจ์จ์ฑ ์ฆ๊ฐ
public class MyCommentUser2 {
//MYCOMMENT ํ
์ด๋ธ(๊ฒ์๊ธ์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
//=> ๊ฐ์ฒด๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ : ํฌํจ๊ด๊ณ
//=> ํฌํจ๊ด๊ณ๊ฐ ์ฑ๋ฆฝ๋๊ธฐ ์ํด์๋ ๋ฐ๋์ ํ๋์ ๊ฐ์ฒด ์ ์ฅ
private MyComment1 comment;
//MYUSER ํ
์ด๋ธ(ํ์์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
//=> ๊ฐ์ฒด๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ : ํฌํจ๊ด๊ณ
//=> ํฌํจ๊ด๊ณ๊ฐ ์ฑ๋ฆฝ๋๊ธฐ ์ํด์๋ ๋ฐ๋์ ํ๋์ ๊ฐ์ฒด ์ ์ฅ
private MyUser user;
public MyCommentUser2() { }
public MyComment1 getComment() {return comment;}
public void setComment(MyComment1 comment) {this.comment = comment;}
public MyUser getUser() {return user;}
public void setUser(MyUser user) {this.user = user;}
}
6) ๐ค MyReply.java
package xyz.itwill.dto;
/*
MYREPLY ํ
์ด๋ธ : ๊ฒ์๊ธ์ ๋ํ ๋๊ธ์ ์ ์ฅํ๊ธฐ ์ํ ํ
์ด๋ธ
create table myreply(reply_no number primary key, reply_id varchar2(50)
, reply_content varchar2(100), reply_date date, reply_comment_no number );
MYREPLY_SEQ ์ํ์ค : MYREPLY ํ
์ด๋ธ์ REPLY_NO ์ปฌ๋ผ์ ์ ์ฅ๋ ์๋ ์ฆ๊ฐ๊ฐ์ ์ ๊ณตํ๊ธฐ ์ํ ์ํ์ค
create sequence myreply_seq;
์ด๋ฆ ๋? ์ ํ
---------------- -------- -------------
REPLY_NO NOT NULL NUMBER - ๋๊ธ ๋ฒํธ : PK์ ์ฝ์กฐ๊ฑด
REPLY_ID VARCHAR2(50) - ๋๊ธ ์์ฑ์(์์ด๋) : FK์ ์ฝ์กฐ๊ฑด - MYUSER ํ
์ด๋ธ์ user_id์ฐธ์กฐ
REPLY_CONTENT VARCHAR2(100) - ๋๊ธ ๋ด์ฉ
REPLY_DATE DATE - ๋๊ธ ์์ฑ์ผ
REPLY_COMMENT_NO NUMBER - ๊ฒ์๊ธ ๋ฒํธ : FK์ ์ฝ์กฐ๊ฑด - MYCOMMENT ํ
์ด๋ธ์ comment_no์ฐธ์กฐ
=> "ํ
์ด๋ธ ์ ๊ทํ"๋ฅผ ํตํด ์๋ชป๋ ๊ฐ์ด ์ ์ฅ๋์ง ์๋๋ก ๋ง๋๋ ๊ฒ์ด ํต์ฌ
=> ์ค์ ํ์ฌ์์๋ FK ์ ์ฝ์กฐ๊ฑด์ ์ ๋ง๋ค์ง ์์
(๋ถ๋ชจํ
์ด๋ธ์ ์ปฌ๋ผ๊ฐ์ด ์์ผ๋ฉด ์ฐธ์กฐ๊ฐ ์๋๋ฏ๋ก ๊ฐ ์ ์ฅ์ด ์๋๊ธฐ ๋๋ฌธ)
*/
public class MyReply {
private int replyNo;
private String replyId;
private String replyContent;
private String replyDate;
private int replyCommentNo;
public MyReply() { }
public int getReplyNo() {return replyNo;}
public void setReplyNo(int replyNo) {this.replyNo = replyNo;}
public String getReplyId() {return replyId;}
public void setReplyId(String replyId) {this.replyId = replyId;}
public String getReplyContent() {return replyContent;}
public void setReplyContent(String replyContent) {this.replyContent = replyContent;}
public String getReplyDate() {return replyDate;}
public void setReplyDate(String replyDate) {this.replyDate = replyDate;}
public int getReplyCommentNo() {return replyCommentNo;}
public void setReplyCommentNo(int replyCommentNo) {this.replyCommentNo = replyCommentNo;}
}
7) ๐ค MyReplyUser.java - ํ๋์ ๊ฐ์ฒด ์ ์ฅ - 1:1 ๊ด๊ณ
package xyz.itwill.dto;
//MYREPLY ํ
์ด๋ธ๊ณผ MYUSER ํ
์ด๋ธ์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
//=> 1:1 ๊ด๊ณ์ ์กฐ์ธ ๊ฒ์ ๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
public class MyReplyUser {
/*
//MYREPLY ํ
์ด๋ธ(๋๊ธ์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
private int replyNo;
private String replyId;
private String replyContent;
private String replyDate;
private int replyCommentNo;
//MYUSER ํ
์ด๋ธ(ํ์์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
private String userId;
private String userName;
*/
//MYREPLY ํ
์ด๋ธ(๋๊ธ์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
private MyReply reply;
//MYUSER ํ
์ด๋ธ(ํ์์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ 1๊ฐ
private MyUser user;
public MyReplyUser() { }
public MyReply getReply() {return reply;}
public void setReply(MyReply reply) {this.reply = reply;}
public MyUser getUser() {return user;}
public void setUser(MyUser user) {this.user = user;}
}
8) ๐คMyCommentReply.java - 1:N๊ด๊ณ
package xyz.itwill.dto;
import java.util.List;
//MYCOMMENT ํ
์ด๋ธ๊ณผ MYREPLY ํ
์ด๋ธ์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
//=> MYCOMMENT๋ ํ๋๋ง ๊ฒ์๋์ง๋ง, MYREPLY๋ ์ฌ๋ฌ๊ฐ ๊ฒ์๋ ์ ์์
// => 1:N ๊ด๊ณ์ ์กฐ์ธ ๊ฒ์ ๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
public class MyCommentReply {
private int commentNo;
//MYCOMMENT ํ
์ด๋ธ(๊ฒ์๊ธ์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ : 1๊ฐ
private MyComment1 comment;
//MYREPLY ํ
์ด๋ธ(๋๊ธ์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ : 0๊ฐ ์ด์
private List<MyReply> replyList;
public MyCommentReply() { }
public int getCommentNo() {return commentNo;}
public void setCommentNo(int commentNo) {this.commentNo = commentNo;}
public MyComment1 getComment() {return comment;}
public void setComment(MyComment1 comment) {this.comment = comment;}
public List<MyReply> getReplyList() {return replyList;}
public void setReplyList(List<MyReply> replyList) {this.replyList = replyList;}
}
9) ๐คMyCommentReplyUser.java - 1 : 1 : N๊ด๊ณ
- 1(MYCOMMENT) : 1(MYUSER)๊ด๊ณ + N(MYREPLY) : 1(MYUSER)๊ด๊ณ
- ์กฐ์ธ 3๊ฐ ๋์ด์์
- ์กฐ์ธ 1 - 1(MYCOMMENT) : 1(MYUSER) comment_id = user=id
- ์กฐ์ธ 2 - N(MYREPLY) : 1(MYUSER) reply_id = user_id
- ์กฐ์ธ 3 - 1(MYCOMMENT) : 1(MYREPLY) comment_no = reply_comment_no
package xyz.itwill.dto;
import java.util.List;
//[MYCOMMENTํ
์ด๋ธ๊ณผ MYUSERํ
์ด๋ธ] ๋ฐ [MYREPLYํ
์ด๋ธ๊ณผ MYUSERํ
์ด๋ธ]์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํด๋์ค
public class MyCommentReplyUser {
//MYCOMMENT ํ
์ด๋ธ(๊ฒ์๊ธ์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ : 1๊ฐ
//=> ์ด์ฐจํผ ๊ฐ์ ์ ์ฅํ๋ ํ๋๊ฐ ๋จผ์ ๋์์ผ ํ๋ฏ๋ก, ๊ฐ์ฒด๋ง๊ณ ๊ฐ์ผ๋ก ์ ์ฅํจ
private int commentNo;
private String commentId;
private String commentContent;
private String commentDate;
//MYUSER ํ
์ด๋ธ(ํ์์ ๋ณด - ๊ฒ์๊ธ)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋ - ๊ฒ์ํ : 1๊ฐ
private MyUser user;
//MYREPLY ํ
์ด๋ธ(๋๊ธ์ ๋ณด)๊ณผ MYUSER ํ
์ด๋ธ(ํ์์ ๋ณด)์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๊ธฐ ์ํ
//ํ๋ - ๊ฒ์ํ : 0๊ฐ ์ด์
//= >MyReplyUser๊ฐ์ฒด ์ด์ฉ : MyReply๊ณผ MyUser๋ฅผ ์ ์ฅํ๋ ๊ฐ์ฒด
private List<MyReplyUser> replyUserList;
public MyCommentReplyUser() { }
public int getCommentNo() {return commentNo;}
public void setCommentNo(int commentNo) {this.commentNo = commentNo;}
public String getCommentId() {return commentId;}
public void setCommentId(String commentId) {this.commentId = commentId;}
public String getCommentContent() {return commentContent;}
public void setCommentContent(String commentContent) {this.commentContent = commentContent;}
public String getCommentDate() {return commentDate;}
public void setCommentDate(String commentDate) {this.commentDate = commentDate;}
public MyUser getUser() {return user;}
public void setUser(MyUser user) {this.user = user;}
public List<MyReplyUser> getReplyUserList() {return replyUserList;}
public void setReplyUserList(List<MyReplyUser> replyUserList) {this.replyUserList = replyUserList;}
}
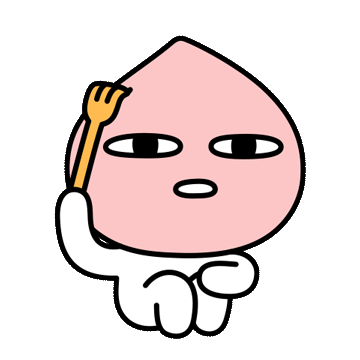
02. Mapper๋ฐ์ธ๋ฉ
1) [Mapper๋ฐ์ธ๋ฉ] MyCommentMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "<https://mybatis.org/dtd/mybatis-3-mapper.dtd>">
<mapper namespace="xyz.itwill.mapper.MyCommentMapper">
<!-- cache : SELECT ๋ช
๋ น์ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์์ ๋ฉ๋ชจ๋ฆฌ(Cache Memory)์ ์ ์ฅํ์ฌ ๋น ๋ฅธ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ
์ ๊ณตํ๊ธฐ ์ํ ์๋ฆฌ๋จผํธ - ๊ฐ๋
์ฑ ์ฆ๊ฐ -->
<!-- => ์ฌ์ฉ์๊ฐ SELECT ๋ช
๋ น์ ์์ฒญํ๋ฉด mybatis๊ฐ DataBase์์ ๊ฒ์ํด์ ๊ทธ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์์ ๋ฉ๋ชจ๋ฆฌ์ ์ ์ฅํ๊ณ ,
๋ค๋ฅธ ์ฌ์ฉ์๊ฐ ๋๊ฐ์ SELECT ๋ช
๋ น์ ์์ฒญํ๋ฉด ์์๋ฉ๋ชจ๋ฆฌ์ ์๋ ๊ฐ์ฒด๋ฅผ ๋ฐ๋ก์ค -->
<!-- => INSERT, UPDATE, DELETE ๋ช
๋ น์ด ์คํ๋๋ฉด ์์๋ฉ๋ชจ๋ฆฌ์ ์ ์ฅ๋ ๊ฒ์๊ฒฐ๊ณผ๋ ์๋ ์ด๊ธฐํ๋จ -->
<!-- => ์ฐ๋ฆฌ๊ฐ ์ฌ์ฉํ๋ ์น์์๋ ๋น๋ฒํ๊ฒ INSERT, UPDATE, DELETE ๋ช
๋ น์ด ์ผ์ด๋๋ฏ๋ก ๊ตณ์ด cache ์๋ฆฌ๋จผํธ ์ธ ์ด์ ๊ฐ ์์ -->
<!-- => ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ์ฅํ๋ ๊ฐ์ฒด๋ ๋ฐ๋์ ๊ฐ์ฒด ์ง๋ ฌํ ํด๋์ค๋ก ์์ฑํด์ผํจ -->
<!-- <cache/> -->
๐MyComment1 ์ด์ฉ : ํ ์ด๋ธ์ ์ปฌ๋ผ๋ช ๊ณผ ํ๋๋ช ์ด ๊ฐ์
- insertComment1
<!-- insert -->
<insert id="insertComment1" parameterType="MyComment1">
insert into mycomment values(mycomment_seq.nextval, #{commentId}, #{commentContent}, sysdate)
</insert>
- insertComment2
<!-- insert : selectKey ์ด์ฉ -->
<insert id="insertComment2" parameterType="MyComment1">
<!-- selectKey : [SELECT ๋ช
๋ น]์ ๊ฒ์ ๊ฒฐ๊ณผ๊ฐ์ insert ์๋ฆฌ๋จผํธ์ parameterType ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋๊ฐ์ผ๋ก ์ ์ฅํ๊ธฐ ์ํ ์๋ฆฌ๋จผํธ -->
<!-- => insert ์๋ฆฌ๋จผํธ์ ์ข
์๋ ์๋ฆฌ๋จผํธ -->
<!-- => jsp1์ DAOํด๋์ค์์ selectNextNum() ๋ฉ์๋์ ๋์ผํ ์ญํ -->
<!-- => ์ผ๋ฐ์ ์ผ๋ก ์๋ ์ฆ๊ฐ๊ฐ(์ซ์) ๋๋ ๋์๊ฐ(๋ฌธ์์ด)์ SELECT ๋ช
๋ น์ผ๋ก ๊ฒ์ํ์ฌ ๊ฐ์ฒด์ ํ๋์ ์ ์ฅํ์ฌ INSERT ๋ช
๋ น์์ ์ฌ์ฉ -->
<!-- => ์ค๋ผํด์ ๋์๊ฐ(๋ฌธ์์ด)์ด ์์ผ๋ฏ๋ก ์ฐ๋ฆฌ๋ ์๋ ์ฆ๊ฐ๊ฐ(์ซ์)์ ์ธ ๋ ์ด์ฉ -->
<!-- => ์๋์ฒ๋ผ ์ค์ ํ๋ฉด ์ด๋ค ์๋์ฆ๊ฐ๊ฐ์ผ๋ก ์ฝ์
๋์๋์ง ๊ทธ ์ซ์๊ฐ ๋ช
ํํ ๋ก๊น
์ ๋ณด๋ฅผ ํตํด ํ์ธ ๊ฐ๋ฅ -->
<!-- ์๋์ฆ๊ฐ๊ฐ ๋ง๋ค๊ธฐ ์ํด ์ฌ์ฉ -->
<!-- ์๋ ์ฆ๊ฐ๊ฐ์ ์ฌ๋ฌ๊ฐ์ ์ปฌ๋ผ์์ ์ด์ฉ ๊ฐ๋ฅ (์ฆ, ์๋์ฆ๊ฐ๊ฐ ์ฌ์ฌ์ฉ ๊ฐ๋ฅ) -->
<!-- ex. ๋ค์ค๋ต๋ณํ ๊ฒ์ํ๋ง๋ค ๋ ์ด์ฉํ๋ฉด ์ข์(num์ปฌ๋ผ๊ฐ๊ณผ ref์ปฌ๋ผ๊ฐ์ ์๋์ฆ๊ฐ๊ฐ ์ด์ฉ)-->
<!-- resultType์์ฑ : SELECT ๋ช
๋ น์ผ๋ก ๊ฒ์๋ ๊ฒฐ๊ณผ๊ฐ์ ๋ฐํ๋ฐ๊ธฐ ์ํ Java ์๋ฃํ์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- keyProperty์์ฑ : insert ์๋ฆฌ๋จผํธ์ parameterType์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋๋ช
์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => SELECT ๋ช
๋ น์ ๊ฒ์ ๊ฒฐ๊ณผ๊ฐ์ ์ ๊ณต๋ฐ์ Java ๊ฐ์ฒด์ ํ๋์ ์ ์ฅํ๊ธฐ ์ํด ์ค์ -->
<!-- order์์ฑ: BEFORE ๋๋ AFTER ์ค ํ๋๋ฅผ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => BEFORE : insert๋ช
๋ น ์คํ ์ select ๋ช
๋ น ๋จผ์ ์คํ-->
<!-- => AFTER : insert๋ช
๋ น ์คํ ํ select ๋ช
๋ น ์คํ-->
<selectKey resultType="int" keyProperty="commentNo" order="BEFORE">
select mycomment_seq.nextval from dual
</selectKey>
insert into mycomment values(#{commentNo}, #{commentId}, #{commentContent}, sysdate)
</insert>
- selectCommentList1
<!-- select - ์๋๋งคํ -->
<!-- resultType ์์ฑ์ ์ฌ์ฉํ์ฌ ์๋ ๋งคํ ์ฒ๋ฆฌ๋ก Java ๊ฐ์ฒด๋ฅผ ์ ๊ณต -->
<!-- => ๊ฒ์ํ์ ์ปฌ๋ผ๋ช
๊ณผ resultType ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋๋ช
์ด ๊ฐ๋๋ก ์์ฑ -->
<select id="selectCommentList1" resultType="MyComment1">
select * from mycomment order by comment_no desc
</select>
๐MyComment2 ์ด์ฉ : ํ ์ด๋ธ์ ์ปฌ๋ผ๋ช ๊ณผ ํ๋๋ช ์ด ๋ค๋ฆ
- selectCommentList2
<!-- select - null ์ ๊ณต -->
<!-- ๊ฒ์ํ์ ์ปฌ๋ผ๋ช
๊ณผ resultType ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋๋ช
์ด ๋ชจ๋ ๋ค๋ฅธ ๊ฒฝ์ฐ
resultType ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ๊ฐ์ฒด ๋์ NULL ์ ๊ณต : NullPoniterException ๋ฐ์-->
<select id="selectCommentList2" resultType="MyComment2">
select * from mycomment order by comment_no desc
</select>
- selectCommentList2
<!-- select - ์๋๋งคํ -->
<!-- ๊ฒ์ํ์ ์ปฌ๋ผ๋ช
์ esultType ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋๋ช
๊ณผ ๊ฐ๋๋ก Column Alias
๊ธฐ๋ฅ์ ์ฌ์ฉํ์ฌ ๊ฒ์ - ์ปฌ๋ผ์ ๋ณ์นญ์ผ๋ก ์ฌ์ฉํ๊ธฐ ๋ถ์ ์ ํ ๋จ์ด๋ "" ๊ธฐํธ๋ฅผ ์ฌ์ฉํ์ฌ ํํ -->
<!-- => date๋ ๋ ์ง์ ์๊ฐ์ ํํํ๋ Java ์๋ฃํ(ํค์๋)์ด๊ธฐ ๋๋ฌธ์ "date"๋ก ํํ ํ์ -->
<select id="selectCommentList2" resultType="MyComment2">
select comment_no no, comment_id id,comment_content content,
comment_date "date" from mycomment order by comment_no desc
</select>
<!-- select - ์๋๋งคํ : Setter๋ฉ์๋๊ฐ ํ๋๊ฐ ์ธํ
-->
<!-- resultMap ์๋ฆฌ๋จผํธ๋ฅผ ์ด์ฉํ์ฌ ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ์ ํด๋์ค์ ํ๋์ ์ ์ฅ๋๋๋ก ์ค์ ํ์ฌ ์ ๊ณต -->
<!-- type์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ Java ํด๋์ค์ [๊ธฐ๋ณธ ์์ฑ์]๋ก ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ , id ์๋ฆฌ๋จผํธ ๋๋
result ์๋ฆฌ๋จผํธ๋ฅผ ์ฌ์ฉํ์ฌ ๊ฐ์ฒด์ [Setter ๋ฉ์๋๋ฅผ ํธ์ถ]ํ์ฌ ํ๋๊ฐ์ด ๋ณ๊ฒฝ๋๋๋ก ์ฒ๋ฆฌ -->
<resultMap type="MyComment2" id="myComment2ResultMap">
<id column="comment_no" property="no"/>
<result column="comment_id" property="id"/>
<result column="comment_content" property="content"/>
<result column="comment_date" property="date"/>
</resultMap>
<!-- select ์๋ฆฌ๋จผํธ์ resultMap ์์ฑ์ ์ฌ์ฉํ์ฌ ๊ฒ์ํ์ Java ๊ฐ์ฒด๋ก ์๋ ๋งคํํ์ฌ ์ ๊ณต -->
<select id="selectCommentList2" resultMap="myComment2ResultMap">
select * from mycomment order by comment_no desc
</select>
<!-- select - ์๋๋งคํ : ๋งค๊ฐ๋ณ์๊ฐ ์๋ ์์ฑ์๊ฐ ํ๋๊ฐ ์ธํ
-->
<!-- constructor ์๋ฆฌ๋จผํธ๋ฅผ ์ฌ์ฉํ์ฌ type์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ๋งค๊ฐ๋ณ์๊ฐ ์์ฑ๋ ์์ฑ์๋ก ๊ฐ์ฒด๋ฅผ ์์ฑํ๊ณ ์์ฑ์ ๋งค๊ฐ๋ณ์์ ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ์ ์ ๋ฌํ์ฌ ๊ฐ์ฒด์ ํ๋์ ์ ์ฅ๋๋๋ก ๋งคํ ์ฒ๋ฆฌ -->
<resultMap type="MyComment2" id="myComment2ConstructResultMap">
<!-- constructor : resultMap ์๋ฆฌ๋จผํธ์ type์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ [์์ฑ์๋ฅผ ์ด์ฉ]ํ์ฌ ๋งคํ ์ฒ๋ฆฌํ๊ธฐ ์ํ ์ ๋ณด๋ฅผ ์ ๊ณตํ๋ ์๋ฆฌ๋จผํธ -->
<!-- => ํ์ ์๋ฆฌ๋จผํธ : idArg, arg ์ค ํ๋ ์ฌ์ฉ-->
<!-- => [์์ฑ์ ๋งค๊ฐ๋ณ์์ ๊ฐฏ์(2๊ฐ)์ ์๋ฃํ]๊ณผ [ํ์ ์๋ฆฌ๋จผํธ์ ๊ฐฏ์(2๊ฐ)์ ์๋ฃํ(javaType)]์ด ๋์ผํด์ผ๋ง ๋งคํ ๊ฐ๋ฅ -->
<constructor>
<!-- idArg : ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ์ ์์ฑ์ ๋งค๊ฐ๋ณ์์ ์ ๋ฌํ๊ธฐ ์ํ ์๋ฆฌ๋จผํธ -->
<!-- => PK ์ ์ฝ์กฐ๊ฑด์ด ์ค์ ๋ ์ปฌ๋ผ๊ฐ์ ์ ๊ณต๋ฐ์ ์์ฑ์ ๋งค๊ฐ๋ณ์์ ์ ๋ฌํ๊ธฐ ์ํด ์ฌ์ฉ -->
<!-- column์์ฑ : ๊ฒ์ํ์ ์ปฌ๋ผ๋ช
์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- javaType์์ฑ : ์์ฑ์ ๋งค๊ฐ๋ณ์์ ์๋ฃํ์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => Java ์๋ฃํ ๋์ typeAlias ์๋ฆฌ๋จผํธ๋ก ์ค์ ๋ ๋ณ์นญ ์ฌ์ฉ ๊ฐ๋ฅ -->
<!-- => ์ฃผ์) ๋ฐ๋์ [ _int : ์์๊ฐ] ๋ก ์์ฑ -->
<idArg column="comment_no" javaType="_int"/>
<!-- arg : ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ์ ์์ฑ์ ๋งค๊ฐ๋ณ์์ ์ ๋ฌํ๊ธฐ ์ํ ์๋ฆฌ๋จผํธ -->
<arg column="comment_id" javaType="string"/>
<!-- 2๊ฐ(no,id)์ ๋งค๊ฐ๋ณ์๋ง ์๋ ์์ฑ์๊ฐ ์๊ธฐ ๋๋ฌธ์ ์๋ 2๊ฐ๋ ์์ด๋ ์๋ฌ ์๋จ์ด์ง๊ณ null๊ฐ์ด ์ ์ฅ๋ ๊ฒ์ -->
<!--
<arg column="comment_content" javaType="string"/>
<arg column="comment_date" javaType="string"/>
-->
</constructor>
<!-- constructor ์๋ฆฌ๋จผํธ์ id์๋ฆฌ๋จผํธ(result ์๋ฆฌ๋จผํธ)๋ฅผ ๊ฐ์ด ์ฌ์ฉํ์ฌ ๋งคํ ์ฒ๋ฆฌ ๊ฐ๋ฅ -->
<result column="comment_content" property="content"/>
<result column="comment_date" property="date"/>
</resultMap>
<select id="selectCommentList2" resultMap="myComment2ConstructResultMap">
select * from mycomment order by comment_no desc
</select>
๐MyComment3 ์ด์ฉ : ํ ์ด๋ธ์ ์ปฌ๋ผ๋ช ๊ณผ ํ๋๋ช ์ด ๋ค๋ฆ - ๋ ๊ฐ์ ํ ์ด๋ธ ์กฐ์ธํ์ฌ ์ ์ฅ๋ ๊ฐ์ฒด
- selectCommentList3
<!-- select - ์๋๋งคํ -->
<select id="selectCommentList3" resultType="MyComment3">
select comment_no "no", comment_id "id", user_name "name",
comment_content "content", comment_date "date" from mycomment
join myuser on comment_id=user_id
order by comment_no desc
</select>
<!-- select - ์๋๋งคํ -->
<resultMap type="MyComment3" id="myComment3ResultMap">
<id column="comment_no" property="no"/>
<result column="comment_id" property="id"/>
<result column="user_name" property="name"/>
<result column="comment_content" property="content"/>
<result column="comment_date" property="date"/>
</resultMap>
<select id="selectCommentList3" resultMap="myComment3ResultMap">
select comment_no, comment_id, user_name, comment_content, comment_date
from mycomment join myuser on comment_id=user_id order by comment_no desc
</select>
๐MyCommentUser1 ์ด์ฉ : ๋ ๊ฐ์ ํ ์ด๋ธ์ ์ปฌ๋ผ๊ฐ ๋ชจ๋๊ฐ ์ ์ฅ๋ ๊ฐ์ฒด
- selectCommentUserList1
<!-- select - ์๋๋งคํ -->
<select id="selectCommentUserList1" resultType="MyCommentUser1">
select comment_no, comment_id, comment_content, comment_date, user_id, user_name
from mycomment join myuser on comment_id=user_id order by comment_no desc
</select>
๐MyCommentUser2 ์ด์ฉ : ๋ ๊ฐ์ ํ ์ด๋ธ์ ์ปฌ๋ผ๊ฐ์ ๊ฐ์ง ๊ฐ์ฒด๊ฐ ์ ์ฅ๋ ๊ฐ์ฒด - 1 : 1 ๊ด๊ณ์ ์กฐ์ธ
- selectCommentUserList2
<!-- select - ๋ฌด์กฐ๊ฑด ์๋๋งคํ๋ง ๊ฐ๋ฅ -->
<!-- resultType ์ฌ์ฉ ๋ถ๊ฐ๋ฅํ๋ฏ๋ก ๋ฐ๋์ resultMap ์ด์ฉ : ์๋๋งคํ๋ง ๊ฐ๋ฅ -->
<!-- ๊ฒ์ํ์ ์ปฌ๋ผ๋ช
๊ณผ ํ๋๋ช
์ด ๋ค๋ฅด๋ฏ๋ก ์ฌ์ฉ ๋ถ๊ฐ๋ฅํ๊ธฐ๋ ํ๊ณ , MyCommentUser2์ ํ๋์๋ ๊ฐ์ด ์๋ ๊ฐ์ฒด๋ฅผ ์ ์ฅํ๋ฏ๋ก ์ง๊ธ๊น์ง ํ๋ ๊ฒ์ฒ๋ผ ์๋ ๋งคํ ๋ชปํจ -->
<!-- =>์ฆ, association ์๋ฆฌ๋จผํธ๊ฐ ํ์ -->
<!-- resultMap ์๋ฆฌ๋จผํธ์ type ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋๊ฐ Java ๊ฐ์ฒด๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋๋ก ์ ์ธ๋ ๊ฒฝ์ฐ ํ์ ์๋ฆฌ๋จผํธ๋ก association ์๋ฆฌ๋จผํธ๋ฅผ ์ฌ์ฉํ์ฌ Java ๊ฐ์ฒด๋ฅผ ์ ๊ณต๋ฐ์ ํ๋์ ์ ์ฅ๋๋๋ก ์ค์ -->
<resultMap type="MyCommentUser2" id="myCommentUser2ResultMap">
<!-- association : 1:1 ๊ด๊ณ์ ํ
์ด๋ธ ์กฐ์ธ์์ 1๊ฐ์ ๊ฒ์ํ์ Java ๊ฐ์ฒด๋ก ์ ๊ณต๋ฐ์ ํ๋์ ์ ์ฅ๋๋๋ก ๋งคํ ์ฒ๋ฆฌํ๋ ์๋ฆฌ๋จผํธ -->
<!-- => id ์๋ฆฌ๋จผํธ์ result ์๋ฆฌ๋จผํธ๋ฅผ ํ์ ์๋ฆฌ๋จผํธ๋ก ์ฌ์ฉํ์ฌ ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ์ด ํฌํจ ๊ด๊ณ์ ๊ฐ์ฒด์ ํ๋์ ์ ์ฅ๋๋๋ก ์ค์ -->
<!-- property ์์ฑ : ํฌํจ ๊ด๊ณ์ ๊ฐ์ฒด๋ฅผ ์ ์ฅํ๊ธฐ ์ํ ํ๋๋ช
์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- javaType ์์ฑ : ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ์ ๊ณต๋ฐ๊ธฐ ์ํ ๊ฐ์ฒด์ Java ์๋ฃํ์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => Java ์๋ฃํ ๋์ typeAlias ์๋ฆฌ๋จผํธ๋ก ์ค์ ๋ ๋ณ์นญ ์ฌ์ฉ ๊ฐ๋ฅ -->
<!-- => ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ๋ค์ ํฌํจ๊ด๊ณ์ ๊ฐ์ฒด์ ํ๋์ ์ ์ฅํ ์ ์๋๋ก ๋ง๋๋ ๊ฒ -->
<!-- MyComment1 ๊ฐ์ฒด๋ฅผ ์ ๊ณต๋ฐ์ comment ํ๋์ ์ ์ฅํด -->
<association property="comment" javaType="MyComment1">
<id column="comment_no" property="commentNo"/>
<result column="comment_id" property="commentId"/>
<result column="comment_content" property="commentContent"/>
<result column="comment_date" property="commentDate"/>
</association>
<!-- MyUser ๊ฐ์ฒด๋ฅผ ์ ๊ณต๋ฐ์ user ํ๋์ ์ ์ฅํด -->
<association property="user" javaType="MyUser">
<id column="user_id" property="userId"/>
<result column="user_name" property="userName"/>
</association>
</resultMap>
<select id="selectCommentUserList2" resultMap="myCommentUser2ResultMap">
select comment_no, comment_id, comment_content, comment_date, user_id, user_name
from mycomment join myuser on comment_id=user_id order by comment_no desc
</select>
๐MyComment1 + ๐คMyReply ์ด์ฉ
- selectComment
<!-- [๐๋ฐฉ๋ฒ1] ์กฐ์ธํ์ง ์๊ณ ๊ฐ๊ฐ์ DAO ๋ง๋ค์ด์ ๋ฉ์๋ ํธ์ถํด ์ถ๋ ฅํ๊ธฐ -->
<!-- ๊ฒ์๊ธ๋ฒํธ๋ฅผ ์ ๋ฌ๋ฐ์ MYCOMMENT ํ
์ด๋ธ์ ์ ์ฅ๋ ํด๋น ๊ฒ์๊ธ๋ฒํธ์ ๊ฒ์๊ธ์ ๊ฒ์ํ์ฌ MyComment1 ๊ฐ์ฒด๋ก ์ ๊ณตํ๋ ์๋ฆฌ๋จผํธ - ๊ฒ์ํ 1๊ฐ -->
<!-- resultType์ฐ๋ ์ด์ : MyComment1ํ
์ด๋ธ์ ์ปฌ๋ผ๋ช
๊ณผ ํ๋๋ช
์ด ๊ฐ๊ธฐ ๋๋ฌธ์ ์๋๋งคํ๋จ -->
<select id="selectComment" parameterType="int" resultType="MyComment1">
select * from mycomment where comment_no=#{commentNo}
</select>
- selectCommentNoReplyList
<!-- ์๋๋ MyReplyMapper.xml ๋ฌธ์์ ์์ด์ผ ํ๋ ๋ถ๋ถ์ด์ง๋ง ๊ทธ๋ฅ MyCommentMapper.xml ๋ฌธ์์ ์์ฑํ ๊ฒ์ -->
<!-- ๊ฒ์๊ธ๋ฒํธ๋ฅผ ์ ๋ฌ๋ฐ์ MYREPLY ํ
์ด๋ธ์ ์ ์ฅ๋ ํด๋น ๊ฒ์๊ธ๋ฒํธ์ ๋๊ธ์ ๊ฒ์ํ์ฌ MyReply ๊ฐ์ฒด๋ก ์ ๊ณตํ๋ ์๋ฆฌ๋จผํธ - ๊ฒ์ํ 0๊ฐ ์ด์ (๋ฌด์กฐ๊ฑด List๋ก ๋ฐํ)-->
<!-- resultType์ฐ๋ ์ด์ : MyReplyํ
์ด๋ธ์ ์ปฌ๋ผ๋ช
๊ณผ ํ๋๋ช
์ด ๊ฐ๊ธฐ ๋๋ฌธ์ ์๋๋งคํ๋จ -->
<select id="selectCommentNoReplyList" parameterType="int" resultType="MyReply">
select * from myreply where reply_comment_no=#{replyCommentNo} order by reply_no desc
</select>
๐คMyCommentReply ์ด์ฉ
- selectCommentReply
<!-- [๐๋ฐฉ๋ฒ2] ์กฐ์ธํด์ DAO ๋ฉ์๋ 1๋ฒ๋ง ํธ์ถํด ์ถ๋ ฅํ๊ธฐ - 1 : N ๊ด๊ณ์ ์กฐ์ธ -->
<!-- resultMap ์๋ฆฌ๋จผํธ์ type ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋ ์๋ฃํ์ด List์ธ ๊ฒฝ์ฐ collection ์๋ฆฌ๋จผํธ๋ฅผ ์ฌ์ฉํ์ฌ List ๊ฐ์ฒด๋ฅผ ์์ฑํ์ฌ ํ๋์ ์ ์ฅ๋๋๋ก ์ค์ -->
<!-- resultMap ์๋ฆฌ๋จผํธ์ ํ์ ์๋ฆฌ๋จผํธ ์์ฑ ์์ -->
<!-- => constructor, id, result, association, collection, discriminator -->
<!-- => association ์๋ฆฌ๋จผํธ๊ฐ resultMap ์๋ฆฌ๋จผํธ์ ์ฒซ๋ฒ์งธ ํ์ ์๋ฆฌ๋จผํธ์ธ ๊ฒฝ์ฐ ๋ด๋ถ์ ์ผ๋ก selectOne ๋ฉ์๋๋ฅผ ์ฌ์ฉํ์ฌ ํ๋์ ๊ฒ์ ํ์ ๋ํ ๊ฐ์ฒด๋ง ์ ๊ณตํ์ฌ ํ๋์ ์ ์ฅ -->
<!-- ๋ฌธ์ ์ ) association ์๋ฆฌ๋จผํธ๊ฐ resultMap ์๋ฆฌ๋จผํธ์ ์ฒซ๋ฒ์งธ ํ์ ์๋ฆฌ๋จผํธ์ธ ๊ฒฝ์ฐ ๋ค์์ ๊ฒ์ํ์ด ๊ฒ์๋ ๊ฒฝ์ฐ TooManyResultException ๋ฐ์ -->
<!-- ํด๊ฒฐ๋ฒ) ๋ค์ํ์ ๊ฒ์ํ ๊ฒฝ์ฐ resultMap ์๋ฆฌ๋จผํธ์ ์ฒซ๋ฒ์งธ ํ์ ์๋ฆฌ๋จผํธ๋ฅผ association ์๋ฆฌ๋จผํธ๊ฐ ์๋ ๋ค๋ฅธ ์๋ฆฌ๋จผํธ๋ก ์ค์ ํ์ฌ ๋งคํ์ฒ๋ฆฌ : id ์๋ฆฌ๋จผํธ ์ถ๊ฐํจ -->
<resultMap type="MyCommentReply" id="myCommentReplyResultMap">
<id column="comment_no" property="commentNo"/>
<!-- association์ selectOne()๋ฉ์๋๋ฅผ ํธ์ถํด ๋งคํ์ฒ๋ฆฌํด์ฃผ๊ณ ๋ค์์ผ๋ก ๋๊น, ๊ทผ๋ฐ collection์ ํ์ด ์ฌ๋ฌ๊ฐ๋ผ selectOne()์ผ๋ก ์ฒ๋ฆฌ ๋ชปํด์ ์๋ฌ ๋ฐ์ํจ -->
<association property="comment" javaType="MyComment1">
<id column="comment_no" property="commentNo"/>
<result column="comment_id" property="commentId"/>
<result column="comment_content" property="commentContent"/>
<result column="comment_date" property="commentDate"/>
</association>
<!-- collection: List ๊ฐ์ฒด๋ฅผ ์์ฑํ์ฌ ํฌํจ ๊ฐ์ฒด ํ๋์ ์ ์ฅํ๊ธฐ ์ํ ์๋ฆฌ๋จผํธ -->
<!-- => 1:N ๊ด๊ณ์ ํ
์ด๋ธ ์กฐ์ธ์์ 0๊ฐ ์ด์์ ํ
์ด๋ธ ๊ฒ์ํ์ List ๊ฐ์ฒด์ ์์๋ก ์ ์ฅํ์ฌ ์์ฑ -->
<!-- => id ์๋ฆฌ๋จผํธ์ result ์๋ฆฌ๋จผํธ๋ฅผ ํ์ ์๋ฆฌ๋จผํธ๋ก ์ฌ์ฉํ์ฌ ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ์ด List ๊ฐ์ฒด์ ์์ ํ๋์ ์ ์ฅ๋๋๋ก ์ค์ -->
<!-- property ์์ฑ : resultMap ์๋ฆฌ๋จผํธ์ type ์์ฑ๊ฐ์ผ๋ก ์ค์ ๋ ํด๋์ค์ ํ๋๋ช
์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- ofType ์์ฑ : List ๊ฐ์ฒด์ ์ ์ฅ๋ ์์์ Java ์๋ฃํ์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => Java ์๋ฃํ ๋์ typeAlias ์๋ฆฌ๋จผํธ๋ก ์ค์ ๋ ๋ณ์นญ ์ฌ์ฉ ๊ฐ๋ฅ -->
<collection property="replyList" ofType="MyReply">
<id column="reply_no" property="replyNo"/>
<result column="reply_id" property="replyId"/>
<result column="reply_content" property="replyContent"/>
<result column="reply_date" property="replyDate"/>
<result column="reply_comment_no" property="replyCommentNo"/>
</collection>
</resultMap>
<!-- 1:N ๊ด๊ณ์ ์กฐ์ธ์ ๋ฐ๋์ OUTER JOIN ์ฌ์ฉํ์ฌ ๊ฒ์ -->
<!-- ์ด๋ ์กฐ์ธ์ ์กฐ๊ฑด์ด ์ฐธ์ธ ๊ฒฝ์ฐ๋ง ์ถ๋ ฅํ๋ฏ๋ก, n=0์ผ ๋(๊ฒ์๊ธ์ด ์์ ๋) ๊ฒ์์ด ๋์ง ์์-->
<!-- => ๋๊ธ์ด ์๋ ๊ฒ์๊ธ์ ๊ฒ์ํ๊ธฐ ์ํด LEFT OUTER JOIN ์ฌ์ฉ -->
<select id="selectCommentReply" parameterType="int" resultMap="myCommentReplyResultMap">
select comment_no, comment_id, comment_content, comment_date,
reply_no, reply_id, reply_content, reply_date, reply_comment_no
from mycomment left join myreply on comment_no=reply_comment_no
where comment_no=#{commentNo} order by reply_no desc
</select>
๐คMyCommentReplyUser ์ด์ฉ
- selectCommentReplyUser
<!-- [๐๋ฐฉ๋ฒ3] ๐ต3๊ฐ ์กฐ์ธํด์ ์ถ๋ ฅํ๊ธฐ - select ๊ตฌ๋ฌธ ์ ์์ฑํ๊ธฐ!! -->
<!-- [๐ต3๊ฐ ์กฐ์ธํ๋ ๋ฐฉ๋ฒ1] -->
<resultMap type="MyCommentReplyUser" id="myCommentReplyUserResultMap">
<!-- 1. ๊ฐ์ผ๋ก ์ ์ฅ -->
<id column="comment_no" property="commentNo"/>
<result column="comment_id" property="commentId"/>
<result column="comment_content" property="commentContent"/>
<result column="comment_date" property="commentDate"/>
<!-- 2. ๊ฐ์ฒด๋ก ์ ์ฅ -->
<association property="user" javaType="MyUser">
<id column="user_id" property="userId"/>
<result column="user_name" property="userName"/>
</association>
<!-- 3. ๋ฆฌ์คํธ๋ก ์ ์ฅ -->
<collection property="replyUserList" ofType="MyReplyUser">
<!-- 3-1. ๋ฆฌ์คํธ์ ์์๊ฐ ๊ฐ์ฒด๋ผ์ association ์ด์ฉ : replyํ๋์ ์ ์ฅ -->
<association property="reply" javaType="MyReply">
<id column="reply_no" property="replyNo"/>
<result column="reply_id" property="replyId"/>
<result column="reply_content" property="replyContent"/>
<result column="reply_date" property="replyDate"/>
<result column="reply_comment_no" property="replyCommentNo"/>
</association>
<!-- 3-2. ๋ฆฌ์คํธ์ ์์๊ฐ ๊ฐ์ฒด๋ผ์ association ์ด์ฉ : userํ๋์ ์ ์ฅ -->
<association property="user" javaType="MyUser">
<id column="reply_user_id" property="userId"/>
<result column="reply_user_name" property="userName"/>
</association>
</collection>
</resultMap>
<select id="selectCommentReplyUser" parameterType="int" resultMap="myCommentReplyUserResultMap">
select comment_no, comment_id, comment_content, comment_date, user_id, user_name,
reply_no, reply_id, reply_content, reply_date, reply_comment_no, reply_user_id, reply_user_name
from mycomment join myuser on comment_id=user_id left join
(select reply_no, reply_id, reply_content, reply_date, reply_comment_no, user_id reply_user_id, user_name reply_user_name
from myreply join myuser on reply_id=user_id) on comment_no=reply_comment_no
where comment_no=#{commentNo} order by reply_no desc
</select>
<!-- [๐ต3๊ฐ ์กฐ์ธํ๋ ๋ฐฉ๋ฒ2] -->
<!-- autoMapping ์์ฑ : false ๋๋ true ์ค ํ๋๋ฅผ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => autoMapping ์์ฑ๊ฐ์ [true]๋ก ์ค์ ํ ๊ฒฝ์ฐ ๊ฒ์ํ์ ์ปฌ๋ผ๋ช
๊ณผ ํ๋๋ช
๊ฐ์ ๊ฒฝ์ฐ ์๋ ๋งคํ ์ฒ๋ฆฌ -->
<resultMap type="MyCommentReplyUser" id="myCommentReplyUserResultMap" autoMapping="true">
<id column="comment_no" property="commentNo"/>
<association property="user" javaType="MyUser" autoMapping="true"/>
<collection property="replyUserList" select="selectReplyUser" column="comment_no"/>
</resultMap>
<resultMap type="MyReplyUser" id="myReplyUserResultMap" autoMapping="true">
<association property="reply" javaType="MyReply" autoMapping="true"/>
<association property="user" javaType="MyUser" autoMapping="true"/>
</resultMap>
<select id="selectReplyUser" parameterType="int" resultMap="myReplyUserResultMap">
select reply_no,reply_id,reply_content,reply_date,reply_comment_no,user_id,user_name
from myreply join myuser on reply_id=user_id where reply_comment_no=#{replyCommentNo} order by reply_no desc
</select>
<select id="selectCommentReplyUser" parameterType="int" resultMap="myCommentReplyUserResultMap">
select comment_no,comment_id,comment_content,comment_date,user_id,user_name
,reply_no,reply_id,reply_content,reply_date,reply_comment_no
from mycomment join myuser on comment_id=user_id left join myreply on comment_no=reply_comment_no
where comment_no=#{commentNo} order by reply_no desc
</select>
</mapper>
2) [Mapper๋ฐ์ธ๋ฉ] MyCommentMapper.java
package xyz.itwill.mapper;
import java.util.List;
import xyz.itwill.dto.MyComment1;
import xyz.itwill.dto.MyComment2;
import xyz.itwill.dto.MyComment3;
import xyz.itwill.dto.MyCommentReply;
import xyz.itwill.dto.MyCommentReplyUser;
import xyz.itwill.dto.MyCommentUser1;
import xyz.itwill.dto.MyCommentUser2;
import xyz.itwill.dto.MyReply;
public interface MyCommentMapper {
int insertComment1(MyComment1 comment);
int insertComment2(MyComment1 comment);
List<MyComment1> selectCommentList1();
List<MyComment2> selectCommentList2();
List<MyComment3> selectCommentList3();
List<MyCommentUser1> selectCommentUserList1();
List<MyCommentUser2> selectCommentUserList2();
//๐๋ฐฉ๋ฒ1์ ์ด์ฉํ๊ธฐ ์ํด
MyComment1 selectComment(int commentNo);
List<MyReply> selectCommentNoReplyList(int replyCommentNo);
//๐๋ฐฉ๋ฒ2๋ฅผ ์ด์ฉํ๊ธฐ ์ํด
MyCommentReply selectCommentReply(int commentNo);
//๐๋ฐฉ๋ฒ3์ ์ด์ฉํ๊ธฐ ์ํด ๐ต3๊ฐ ์กฐ์ธ
MyCommentReplyUser selectCommentReplyUser(int commentNo);
}
3) [Mapper๋ฐ์ธ๋ฉ] MyReplyMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "<https://mybatis.org/dtd/mybatis-3-mapper.dtd>">
<mapper namespace="xyz.itwill.mapper.MyReplyMapper">
๐คMyReply ์ด์ฉ
- insertReply
<!-- insert : MyReply ์ฝ์
-->
<insert id="insertReply" parameterType="MyReply">
<selectKey resultType="int" keyProperty="replyNo" order="BEFORE">
select myreply_seq.nextval from dual
</selectKey>
insert into myreply values(#{replyNo}, #{replyId}, #{replyContent}, sysdate, #{replyCommentNo})
</insert>
- selectReplyList
<!-- select : MyReply ์ ์ฒด ์ถ๋ ฅ -->
<select id="selectReplyList" resultType="MyReply">
select * from myreply order by reply_no desc
</select>
- selectCountReplyList
<!-- select : MyReply ์ต๊ทผ 5๊ฐ๋ง ์ถ๋ ฅ -->
<!-- MYREPLY ํ
์ด๋ธ์ ์ ์ฅ๋ ๋ชจ๋ ๋๊ธ ์ค์์ ๊ฐ์ฅ ์ต๊ทผ์ ์์ฑ๋ 5๊ฐ์ ๋๊ธ๋ง ๊ฒ์ -->
<!-- ๋ฌธ์ ์ ) XML ๊ธฐ๋ฐ์ ๋งคํผ ํ์ผ์์๋ SQL ๋ช
๋ น์ด ๋ฑ๋ก๋ ์๋ฆฌ๋จผํธ์์ ๊ด๊ณ ์ฐ์ฐ์(> ๋๋ <)๋ฅผ
์ฌ์ฉํ ๊ฒฝ์ฐ ์๋ฆฌ๋จผํธ๋ฅผ ํ์ํ๋ ๊ธฐํธ๋ก ์ธ์๋์ด ์๋ฌ ๋ฐ์ํจ -->
<!-- ํด๊ฒฐ๋ฒ-1) ๊ด๊ณ ์ฐ์ฐ์(> ๋๋ <)๋ฅผ ์ํฐํฐ ๋ ํผ๋ฐ์ค(ํํผ๋ฌธ์)๋ก ๋ณ๊ฒฝํ์ฌ SQL ๋ช
๋ น ์์ฑ -->
<!-- ํด๊ฒฐ๋ฒ-2) CDATA ์ธ์
์ ์ฌ์ฉํ์ฌ SQL ๋ช
๋ น ์์ฑ : CDATA ์์ ์์ฑ๋ ๊ฐ๋ค์ ๋ชจ๋ ๋ฌธ์๊ฐ์ผ๋ก ์ทจ๊ธํ๊ธฐ ๋๋ฌธ -->
<select id="selectCountReplyList" resultType="MyReply">
<!--
select rownum, reply.* from (select * from myreply
order by reply_no desc) reply where rownum <= 5
-->
<![CDATA[
select rownum, reply.* from (select * from myreply
order by reply_no desc) reply where rownum <= 5
]]>
</select>
๐คMyReplyUser ์ด์ฉ
- selectReplyUserList1
<!-- select - ์๋๋งคํ : JOIN ์ด์ฉํจ : MyReplyUser (๊ฐ์ฒด๊ฐ ์ ์ฅ๋ replyํ๋+ userํ๋) ์ถ๋ ฅ -->
<resultMap type="MyReplyUser" id="MyReplyUserResultMap1">
<association property="reply" javaType="MyReply">
<id column="reply_no" property="replyNo"/>
<result column="reply_id" property="replyId"/>
<result column="reply_content" property="replyContent"/>
<result column="reply_date" property="replyDate"/>
<result column="reply_comment_no" property="replyCommentNo"/>
</association>
<association property="user" javaType="MyUser">
<id column="user_id" property="userId"/>
<result column="user_name" property="userName"/>
</association>
</resultMap>
<select id="selectReplyUserList1" resultMap="MyReplyUserResultMap1">
select reply_no, reply_id, reply_content, reply_date, reply_comment_no,
user_id, user_name from myreply join myuser on reply_id=user_id order by reply_no desc
</select>
- selectMyUser
<!-- select - ์๋๋งคํ : JOIN ์ด์ฉ์ํจ -->
<!-- ์กฐ์ธ์ ์ฌ์ฉํ์ง ์๊ณ ๋ (MYREPLY ํ
์ด๋ธ๋ง ๊ฒ์ํด๋) MYUSER ํ
์ด๋ธ์ ๊ฐ๊น์ง ๊ฐ์ด ์ถ๋ ฅํ๋ ๋ฐฉ๋ฒ -->
<!-- => 3.2๋ 3.4 ๋ฒ์ ์ ์ฌ์ฉ ๋ชปํจ -->
<resultMap type="MyReply" id="MyReplyResultMap">
<id column="reply_no" property="replyNo"/>
<result column="reply_id" property="replyId"/>
<result column="reply_content" property="replyContent"/>
<result column="reply_date" property="replyDate"/>
<result column="reply_comment_no" property="replyCommentNo"/>
</resultMap>
<!-- => MyReplyUser๊ฐ์ฒด์๋ MyUser์ MyReply ๋ ๋ค ์ ์ฅ๋ ๊ฒ -->
<resultMap type="MyReplyUser" id="MyReplyUserResultMap2">
<!-- resultMap์์ฑ : resultMap ์๋ฆฌ๋จผํธ์ ์๋ณ์๋ฅผ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => resultMap ์๋ฆฌ๋จผํธ๋ก ์ค์ ๋ ๋งคํ์ ๋ณด๋ฅผ ์ด์ฉํ์ฌ ๊ฒ์ํ์ ์ปฌ๋ผ๊ฐ์ ๊ฐ์ฒด ํ๋์ ์ ์ฅํ์ฌ ๊ฐ์ฒด๋ฅผ ์ ๊ณต๋ฐ์ ํ๋์ ์ ์ฅ - javaType ์์ฑ๊ฐ ์๋ต๊ฐ๋ฅ -->
<!-- => resultMap ์์ฑ์ ์ด์ฉํด ๋ค๋ฅธ resultMap ์๋ฆฌ๋จผํธ๋ฅผ ๊ฐ์ ธ๋ค ๊ฐ์ฒด๋ฅผ ๋ง๋ค์ด ํ๋์ ์ ์ฅ -->
<!-- => resultMap์ ์ฌ์ฌ์ฉ --->
<!-- => replyํ๋์๋ MyReply๊ฐ์ฒด๊ฐ ์ ์ฅ๋จ -->
<association property="reply" resultMap="MyReplyResultMap"/>
<!-- select์์ฑ : select ์๋ฆฌ๋จผํธ์ ์๋ณ์๋ฅผ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => select ์๋ฆฌ๋จผํธ์ SELECT ๋ช
๋ น์ผ๋ก ์คํ๋ ๊ฒ์๊ฒฐ๊ณผ๋ฅผ ๊ฐ์ฒด๋ก ์ ๊ณต๋ฐ์ ํฌํจ ๊ฐ์ฒด์ ํ๋์ ์ ์ฅ -->
<!-- column์์ฑ : SELECT ๋ช
๋ น์ผ๋ก ๊ฒ์๋ ํ์ ์ปฌ๋ผ๋ช
์ ์์ฑ๊ฐ์ผ๋ก ์ค์ -->
<!-- => SELECT ๋ช
๋ น์ผ๋ก ๊ฒ์๋ ํ์ ์ปฌ๋ผ๊ฐ์ select ์์ฑ์ผ๋ก ์ค์ ๋ select ์๋ฆฌ๋จผํธ์ parameterType ์์ฑ๊ฐ์ผ๋ก ์ ๋ฌํ์ฌ SQL ๋ช
๋ น์์ ์ฌ์ฉ -->
<!-- => ์ ๋ฌํด์ select๋ช
๋ น ์คํํด! ๊ทธ๋ฆฌ๊ณ userํ๋์ ์ ์ฅํด!-->
<!-- => userํ๋์๋ MyUser๊ฐ์ฒด๊ฐ ์ ์ฅ๋จ-->
<association property="user" select="selectMyUser" column="reply_id"/>
</resultMap>
<!-- association ์๋ฆฌ๋จผํธ์์๋ง ์ฌ์ฉํ๊ธฐ ์ํ SELECT ๋ช
๋ น ๋ฑ๋ก -->
<!-- => ์ฃผ์) parameterType ์์ฑ์ผ๋ก ์ ๋ฌ๋ฐ์ ๊ฐ์ผ๋ก ๋จ์ผํ์ด ๊ฒ์๋๋๋ก SELECT ๋ช
๋ น ์์ฑ -->
<!-- => DAO ํด๋์ค์ ๋ฉ์๋์์ ์ฌ์ฉ๋ SQL ๋ช
๋ น์ด ์๋๋ฏ๋ก ์ธํฐํ์ด์ค ๊ธฐ๋ฐ์ ๋งคํผ ํ์ผ์์ ์ถ์๋ฉ์๋๋ฅผ ๋ฏธ์ ์ธํจ -->
<!-- select ๋ช
๋ น์ด ์คํ๋๋ฉด ๊ฒ์ํ์ด myuser๊ฐ์ฒด๋ก ๋ง๋ค์ด์ค -->
<select id="selectMyUser" parameterType="string" resultType="MyUser">
select * from myuser where user_id=#{userId} <!-- ๋จ์ผํ๋ง ๊ฒ์๋๋๋ก, ๋ค์คํ์ ์๋จ -->
</select>
- selectReplyUserList2
<!-- ํ
์ด๋ธ ์กฐ์ธ์ ์ฌ์ฉํ์ง ์๊ณ resultMap ์๋ฆฌ๋จผํธ์ ๋งคํ์ ๋ณด๋ฅผ ์ ๊ณต๋ฐ์ 2๊ฐ ์ด์์ ํ
์ด๋ธ์ ์ปฌ๋ผ๊ฐ์ ๊ฒ์ํ์ฌ Java ๊ฐ์ฒด๋ก ์ ๊ณต ๊ฐ๋ฅ -->
<select id="selectReplyUserList2" resultMap="MyReplyUserResultMap2">
select * from myreply order by reply_no desc
</select>
</mapper>
4)[Mapper๋ฐ์ธ๋ฉ] MyReplyMapper.java
package xyz.itwill.mapper;
import java.util.List;
import xyz.itwill.dto.MyReply;
import xyz.itwill.dto.MyReplyUser;
public interface MyReplyMapper {
int insertReply(MyReply reply);
List<MyReply> selectReplyList();
List<MyReply> selectCountReplyList();
List<MyReplyUser> selectReplyUserList1();
List<MyReplyUser> selectReplyUserList2();
}
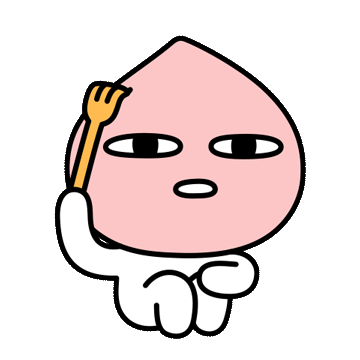
03. SQL๋ฌธ
select comment_no, comment_id, comment_content, comment_date, user_id, user_name,
reply_no, reply_id, reply_content, reply_date, reply_comment_no, reply_user_id, reply_user_name
from mycomment join myuser on comment_id=user_id left join
(select reply_no, reply_id, reply_content, reply_date, reply_comment_no, user_id reply_user_id, user_name reply_user_name
from myreply join myuser on reply_id=user_id) on comment_no=reply_comment_no
where comment_no=1 order by reply_no desc;
1) STEP1 (1:1 ๊ด๊ณ์ ์กฐ์ธ)
- mycomment join myuser on comment_id = user_id
select comment_no, comment_id, comment_content, comment_date,
user_id, user_name
from mycomment join myuser on comment_id=user_id;
2) STEP2 (1:1 ๊ด๊ณ์ ์กฐ์ธ)
- myreply join myuser on reply_id = user_id
select reply_no, reply_id, reply_content, reply_date, reply_comment_no,
user_id reply_user_id, user_name reply_user_name
from myreply join myuser on reply_id=user_id
3) STEP3 (1:N ๊ด๊ณ์ ์กฐ์ธ)
- mycomment join myuser join myreply join myuser on comment_no = reply_comment_no
select comment_no, comment_id, comment_content, comment_date,
user_id, user_name,
reply_no, reply_id, reply_content, reply_date, reply_comment_no,
reply_user_id, reply_user_name
from mycomment join myuser on comment_id=user_id
left join
(select reply_no, reply_id, reply_content, reply_date, reply_comment_no, user_id reply_user_id, user_name reply_user_name
from myreply join myuser on reply_id=user_id)
on comment_no=reply_comment_no
where comment_no=1 order by reply_no desc;
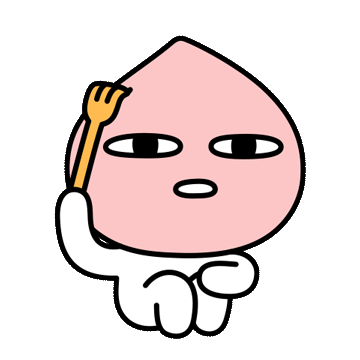
04. DAO
1) MyCommentDAO.java
package xyz.itwill.dao;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import xyz.itwill.dto.MyComment1;
import xyz.itwill.dto.MyComment2;
import xyz.itwill.dto.MyComment3;
import xyz.itwill.mapper.MyCommentMapper;
public class MyCommentDAO extends AbstractSession{
private static MyCommentDAO _dao;
private MyCommentDAO() {
// TODO Auto-generated constructor stub
}
static {
_dao = new MyCommentDAO();
}
public static MyCommentDAO getDAO() {
return _dao;
}
//๐insertComment1
public int insertComment1(MyComment1 commment) {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).insertComment1(commment);
} finally {
sqlSession.close();
}
}
//๐insertComment2
public int insertComment2(MyComment1 commment) {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).insertComment2(commment);
} finally {
sqlSession.close();
}
}
//๐selectCommentList1
public List<MyComment1> selectCommentList1() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentList1();
} finally {
sqlSession.close();
}
}
//๐selectCommentList2
public List<MyComment2> selectCommentList2() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentList2();
} finally {
sqlSession.close();
}
}
//๐selectCommentList3
public List<MyComment3> selectCommentList3() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentList3();
} finally {
sqlSession.close();
}
}
//๐selectCommentUserList1
public List<MyCommentUser1> selectCommentUserList1() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentUserList1();
} finally {
sqlSession.close();
}
}
//๐selectCommentUserList2
public List<MyCommentUser2> selectCommentUserList2() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentUserList2();
} finally {
sqlSession.close();
}
}
//๐MyComment1 + ๐คMyReply : ๋ฉ์๋ 2๊ฐ ๋ง๋ค์ด ๊ฐ๊ฐ ํธ์ถ
//selectComment
public MyComment1 selectComment(int commentNo) {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectComment(commentNo);
} finally {
sqlSession.close();
}
}
//selectCommentNoReplyList
public List<MyReply> selectCommentNoReplyList(int replyCommentNo) {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentNoReplyList(replyCommentNo);
} finally {
sqlSession.close();
}
}
//๐คMyCommentReply : 2๊ฐ ์กฐ์ธํ ๋ฉ์๋ - ์กฐ์ธํ๊ธฐ ๋๋ฌธ์ ๋ฉ์๋ 1๊ฐ ๋ง๋ค์ด ํธ์ถ
public MyCommentReply selectCommentReply(int commentNo) {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentReply(commentNo);
} finally {
sqlSession.close();
}
}
//๐คMyCommentReplyUser : 3๊ฐ ์กฐ์ธํ ๋ฉ์๋
public MyCommentReplyUser selectCommentReplyUser(int commentNo) {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyCommentMapper.class).selectCommentReplyUser(commentNo);
} finally {
sqlSession.close();
}
}
}
2) MyReplyDAO.java
package xyz.itwill.dao;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import xyz.itwill.dto.MyReply;
import xyz.itwill.dto.MyReplyUser;
import xyz.itwill.mapper.MyReplyMapper;
public class MyReplyDAO extends AbstractSession{
private static MyReplyDAO _dao;
private MyReplyDAO() {
// TODO Auto-generated constructor stub
}
static {
_dao = new MyReplyDAO();
}
public static MyReplyDAO getDAO() {
return _dao;
}
//๐คinsertReply
public int insertReply(MyReply reply) {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyReplyMapper.class).insertReply(reply);
} finally {
sqlSession.close();
}
}
//๐คselectReplyList
public List<MyReply> selectReplyList() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyReplyMapper.class).selectReplyList();
} finally {
sqlSession.close();
}
}
//๐คselectCountReplyList
public List<MyReply> selectCountReplyList() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyReplyMapper.class).selectCountReplyList();
} finally {
sqlSession.close();
}
}
//๐คselectReplyUserList1
public List<MyReplyUser> selectReplyUserList1() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyReplyMapper.class).selectReplyUserList1();
} finally {
sqlSession.close();
}
}
//๐คselectReplyUserList2
public List<MyReplyUser> selectReplyUserList2() {
SqlSession sqlSession=getSqlSessionFactory().openSession(true);
try {
return sqlSession.getMapper(MyReplyMapper.class).selectReplyUserList2();
} finally {
sqlSession.close();
}
}
}
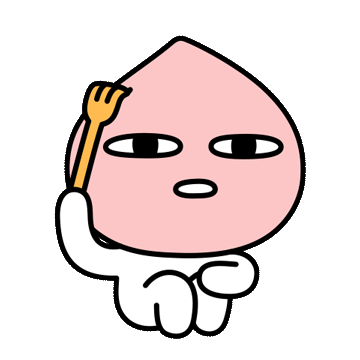
05. ์คํํ JSP
1) [ํ ์คํธ ํ๋ก๊ทธ๋จ] ๐commentInsert1.jsp - comment1 ์ฝ์
ํ ์คํธ ํ๋ก๊ทธ๋จ ๋์ฒด
<%
MyComment1 comment1 = new MyComment1();
comment1.setCommentId("aaa");
comment1.setCommentContent("์ฒซ๋ฒ์งธ ๊ฒ์๊ธ์
๋๋ค.");
MyCommentDAO.getDAO().insertComment1(comment1);
MyComment1 comment2 = new MyComment1();
comment2.setCommentId("xyz");
comment2.setCommentContent("๋๋ฒ์งธ ๊ฒ์๊ธ์
๋๋ค.");
MyCommentDAO.getDAO().insertComment1(comment2);
%>
2) [ํ ์คํธ ํ๋ก๊ทธ๋จ] ๐commentInsert2.jsp - comment1 ์ฝ์
ํ ์คํธ ํ๋ก๊ทธ๋จ ๋์ฒด
<%
MyComment1 comment3 = new MyComment1();
comment3.setCommentId("opq");
comment3.setCommentContent("์ธ๋ฒ์งธ ๊ฒ์๊ธ์
๋๋ค.");
MyCommentDAO.getDAO().insertComment2(comment3);
MyComment1 comment4 = new MyComment1();
comment4.setCommentId("aaa");
comment4.setCommentContent("๋ค๋ฒ์งธ ๊ฒ์๊ธ์
๋๋ค.");
MyCommentDAO.getDAO().insertComment2(comment4);
%>
3) ๐commentListSelect1.jsp - comment1 ์ถ๋ ฅ
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@page import="xyz.itwill.dto.MyComment1"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyComment1> commentList=MyCommentDAO.getDAO().selectCommentList1();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
</style>
</head>
<body>
<h1>๊ฒ์๊ธ ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๊ฒ์๊ธ๋ฒํธ</td>
<td class="name">๊ฒ์๊ธ์์ฑ์</td>
<td class="content">๊ฒ์๊ธ๋ด์ฉ</td>
<td class="date">๊ฒ์๊ธ์์ฑ์ผ</td>
</tr>
<% for(MyComment1 comment:commentList) { %>
<tr>
<td><%=comment.getCommentNo() %></td>
<td><%=comment.getCommentId() %></td>
<td><%=comment.getCommentContent() %></td>
<td><%=comment.getCommentDate() %></td>
</tr>
<% } %>
</table>
</body>
</html>
4) ๐commentListSelect2.jsp - comment2 ์ถ๋ ฅ
<%@page import="xyz.itwill.dto.MyComment2"%>
<%@page import="java.util.List"%>
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyComment2> commentList=MyCommentDAO.getDAO().selectCommentList2();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
</style>
</head>
<body>
<h1>๊ฒ์๊ธ ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๊ฒ์๊ธ๋ฒํธ</td>
<td class="name">๊ฒ์๊ธ์์ฑ์</td>
<td class="content">๊ฒ์๊ธ๋ด์ฉ</td>
<td class="date">๊ฒ์๊ธ์์ฑ์ผ</td>
</tr>
<% for(MyComment2 comment:commentList) { %>
<tr>
<td><%=comment.getNo() %></td>
<td><%=comment.getId() %></td>
<td><%=comment.getContent() %></td>
<td><%=comment.getDate() %></td>
</tr>
<% } %>
</table>
</body>
</html>
5) ๐commentListSelect3.jsp - comment3 ์ถ๋ ฅ
<%@page import="xyz.itwill.dto.MyComment3"%>
<%@page import="java.util.List"%>
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyComment3> commentList=MyCommentDAO.getDAO().selectCommentList3();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
</style>
</head>
<body>
<h1>๊ฒ์๊ธ ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๊ฒ์๊ธ๋ฒํธ</td>
<td class="name">๊ฒ์๊ธ์์ฑ์</td>
<td class="content">๊ฒ์๊ธ๋ด์ฉ</td>
<td class="date">๊ฒ์๊ธ์์ฑ์ผ</td>
</tr>
<% for(MyComment3 comment:commentList) { %>
<tr>
<td><%=comment.getNo() %></td>
<td><%=comment.getName() %> (<%=comment.getId() %>)</td>
<td><%=comment.getContent() %></td>
<td><%=comment.getDate() %></td>
</tr>
<% } %>
</table>
</body>
</html>
6) ๐commentUserListSelect1.jsp - MyCommentUser1 ์ถ๋ ฅ
<%@page import="xyz.itwill.dto.MyCommentUser1"%>
<%@page import="xyz.itwill.dto.MyComment3"%>
<%@page import="java.util.List"%>
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyCommentUser1> commentUserList=MyCommentDAO.getDAO().selectCommentUserList1();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
</style>
</head>
<body>
<h1>๊ฒ์๊ธ ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๊ฒ์๊ธ๋ฒํธ</td>
<td class="name">๊ฒ์๊ธ์์ฑ์</td>
<td class="content">๊ฒ์๊ธ๋ด์ฉ</td>
<td class="date">๊ฒ์๊ธ์์ฑ์ผ</td>
</tr>
<% for(MyCommentUser1 commentUser :commentUserList) { %>
<tr>
<td><%=commentUser.getCommentNo() %></td>
<!-- <td><%=commentUser.getUserName() %> (<%=commentUser.getCommentId() %>)</td> -->
<td><%=commentUser.getUserName() %> (<%=commentUser.getUserId() %>)</td>
<td><%=commentUser.getCommentContent() %></td>
<td><%=commentUser.getCommentDate() %></td>
</tr>
<% } %>
</table>
</body>
</html>
7) ๐commentUserListSelect2.jsp - MyCommentUser2 ์ถ๋ ฅ
aํ๊ทธ๋ก ๋งํฌ ๋ง๋ค๊ธฐ
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@page import="xyz.itwill.dto.MyCommentUser2"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyCommentUser2> commentUserList=MyCommentDAO.getDAO().selectCommentUserList2();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
</style>
</head>
<body>
<h1>๊ฒ์๊ธ ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๊ฒ์๊ธ๋ฒํธ</td>
<td class="name">๊ฒ์๊ธ์์ฑ์</td>
<td class="content">๊ฒ์๊ธ๋ด์ฉ</td>
<td class="date">๊ฒ์๊ธ์์ฑ์ผ</td>
</tr>
<% for(MyCommentUser2 commentUser :commentUserList) { %>
<tr>
<td><%=commentUser.getComment().getCommentNo() %></td>
//<%-- <td><%=commentUser.getUser().getUserName() %> (<%=commentUser.getComment().getCommentId() %>)</td> --%>
<td><%=commentUser.getUser().getUserName() %> (<%=commentUser.getUser().getUserId() %>)</td>
//<%-- <td><%=commentUser.getComment().getCommentContent() %></td> --%>
<td>
//<%--<%=commentUser.getComment().getCommentContent() %>--%>
//<%--<a href="commentReplySelect1.jsp?commentNo=<%=commentUser.getComment().getCommentNo()%>"><%=commentUser.getComment().getCommentContent() %></a> --%>
//<%--<a href="commentReplySelect2.jsp?commentNo=<%=commentUser.getComment().getCommentNo()%>"><%=commentUser.getComment().getCommentContent() %></a> --%>
<a href="commentReplyUserSelect.jsp?commentNo=<%=commentUser.getComment().getCommentNo()%>"><%=commentUser.getComment().getCommentContent() %></a>
</td>
<td><%=commentUser.getComment().getCommentDate() %></td>
</tr>
<% } %>
</table>
</body>
</html>
8) [ํ ์คํธ ํ๋ก๊ทธ๋จ] ๐คReplyInsert.jsp - MyReply ์ฝ์
<%@page import="xyz.itwill.dao.MyReplyDAO"%>
<%@page import="xyz.itwill.dto.MyReply"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
//<%-- ์๋๋ ํ
์คํธ ํ๋ก๊ทธ๋จ์ผ๋ก ๋ง๋ค์ด ํ
์คํธํ๋ ๊ฒ์ด์ง๋ง, ์์ง ๋ฐฐ์ฐ์ง ์์์ผ๋ ์ฐ๋ฆฌ๋ JSP๋ก ๋ง๋ค์ด์ ์ฌ์ฉ --%>
//<%-- ์๋ชป๋ ๊ฒฐ๊ณผ๊ฐ ๋์ฌ ๊ฒฝ์ฐ
// drop sequence myreply_seq;
// create sequence myreply_seq;
// truncate table myreply;
// ํ JSP๋ฌธ์ ์ฌ์คํ
//--%>
<%
//์ฒซ๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ ์ฝ์
- 3๊ฐ
MyReply reply1 = new MyReply();
reply1.setReplyCommentNo(1);
reply1.setReplyId("xyz");
reply1.setReplyContent("์ฒซ๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ-1");
MyReplyDAO.getDAO().insertReply(reply1);
MyReply reply2 = new MyReply();
reply2.setReplyCommentNo(1);
reply2.setReplyId("opq");
reply2.setReplyContent("์ฒซ๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ-2");
MyReplyDAO.getDAO().insertReply(reply2);
MyReply reply3 = new MyReply();
reply3.setReplyCommentNo(1);
reply3.setReplyId("xyz");
reply3.setReplyContent("์ฒซ๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ-3");
MyReplyDAO.getDAO().insertReply(reply3);
//๋๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ ์ฝ์
- 0๊ฐ
//์ธ๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ ์ฝ์
- 1๊ฐ
MyReply reply4 = new MyReply();
reply4.setReplyCommentNo(3);
reply4.setReplyId("xyz");
reply4.setReplyContent("์ธ๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ-1");
MyReplyDAO.getDAO().insertReply(reply4);
//๋ค๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ ์ฝ์
- 2๊ฐ
MyReply reply5 = new MyReply();
reply5.setReplyCommentNo(4);
reply5.setReplyId("xyz");
reply5.setReplyContent("๋ค๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ-1");
MyReplyDAO.getDAO().insertReply(reply5);
MyReply reply6 = new MyReply();
reply6.setReplyCommentNo(4);
reply6.setReplyId("opq");
reply6.setReplyContent("๋ค๋ฒ์งธ ๊ฒ์๊ธ์ ๋ํ ๋๊ธ-2");
MyReplyDAO.getDAO().insertReply(reply6);
%>
9) ๐คreplyListSelect.jsp - MyReply ์ ์ฒด ์ถ๋ ฅ
<%@page import="xyz.itwill.dao.MyReplyDAO"%>
<%@page import="xyz.itwill.dto.MyReply"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyReply> replyList = MyReplyDAO.getDAO().selectReplyList();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
.comment{ width: 100px; }
</style>
</head>
<body>
<h1>๋๊ธ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๋๊ธ๋ฒํธ</td>
<td class="name">๋๊ธ์์ฑ์</td>
<td class="content">๋๊ธ๋ด์ฉ</td>
<td class="date">๋๊ธ์์ฑ์ผ</td>
<td class="comment">๊ฒ์๊ธ๋ฒํธ</td>
</tr>
<% for(MyReply reply:replyList) { %>
<tr>
<td><%=reply.getReplyNo() %></td>
<td><%=reply.getReplyId() %></td>
<td><%=reply.getReplyContent() %></td>
<td><%=reply.getReplyDate() %></td>
<td><%=reply.getReplyCommentNo() %></td>
</tr>
<% } %>
</table>
</body>
</html>
10) ๐คreplyCountListSelect.jsp - MyReply ์ต์ ๊ธ 5๊ฐ๋ง ์ถ๋ ฅ
<%@page import="xyz.itwill.dao.MyReplyDAO"%>
<%@page import="xyz.itwill.dto.MyReply"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyReply> replyList = MyReplyDAO.getDAO().selectCountReplyList();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
.comment{ width: 100px; }
</style>
</head>
<body>
<h1>๋๊ธ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๋๊ธ๋ฒํธ</td>
<td class="name">๋๊ธ์์ฑ์</td>
<td class="content">๋๊ธ๋ด์ฉ</td>
<td class="date">๋๊ธ์์ฑ์ผ</td>
<td class="comment">๊ฒ์๊ธ๋ฒํธ</td>
</tr>
<% for(MyReply reply:replyList) { %>
<tr>
<td><%=reply.getReplyNo() %></td>
<td><%=reply.getReplyId() %></td>
<td><%=reply.getReplyContent() %></td>
<td><%=reply.getReplyDate() %></td>
<td><%=reply.getReplyCommentNo() %></td>
</tr>
<% } %>
</table>
</body>
</html>
11) ๐คreplyUserListSelect1.jsp - MyReplyUser ์ถ๋ ฅ
<%@page import="xyz.itwill.dao.MyReplyDAO"%>
<%@page import="xyz.itwill.dto.MyReplyUser"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyReplyUser> replyUserList = MyReplyDAO.getDAO().selectReplyUserList();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
.comment{ width: 100px; }
</style>
</head>
<body>
<h1>๋๊ธ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๋๊ธ๋ฒํธ</td>
<td class="name">๋๊ธ์์ฑ์</td>
<td class="content">๋๊ธ๋ด์ฉ</td>
<td class="date">๋๊ธ์์ฑ์ผ</td>
<td class="comment">๊ฒ์๊ธ๋ฒํธ</td>
</tr>
<% for(MyReplyUser replyUser:replyUserList) { %>
<tr>
<td><%=replyUser.getReply().getReplyNo() %></td>
<!-- <td><%=replyUser.getUser().getUserName() %> (<%=replyUser.getReply().getReplyId() %>)</td>-->
<td><%=replyUser.getUser().getUserName() %> (<%=replyUser.getUser().getUserId() %>)</td>
<td><%=replyUser.getReply().getReplyContent() %></td>
<td><%=replyUser.getReply().getReplyDate() %></td>
<td><%=replyUser.getReply().getReplyCommentNo() %></td>
</tr>
<% } %>
</table>
</body>
</html>
12) ๐คreplyUserListSelect2.jsp - MyReplyUser ์ถ๋ ฅ
<%@page import="xyz.itwill.dao.MyReplyDAO"%>
<%@page import="xyz.itwill.dto.MyReplyUser"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
List<MyReplyUser> replyUserList = MyReplyDAO.getDAO().selectReplyUserList2();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table {
border: 1px solid black;
border-collapse: collapse;
}
td {
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no { width: 100px; }
.name { width: 150px; }
.content { width: 250px; }
.date { width: 200px; }
.comment{ width: 100px; }
</style>
</head>
<body>
<h1>๋๊ธ๋ชฉ๋ก</h1>
<hr>
<table>
<tr>
<td class="no">๋๊ธ๋ฒํธ</td>
<td class="name">๋๊ธ์์ฑ์</td>
<td class="content">๋๊ธ๋ด์ฉ</td>
<td class="date">๋๊ธ์์ฑ์ผ</td>
<td class="comment">๊ฒ์๊ธ๋ฒํธ</td>
</tr>
<% for(MyReplyUser replyUser:replyUserList) { %>
<tr>
<td><%=replyUser.getReply().getReplyNo() %></td>
<!-- <td><%=replyUser.getUser().getUserName() %> (<%=replyUser.getReply().getReplyId() %>)</td>-->
<td><%=replyUser.getUser().getUserName() %> (<%=replyUser.getUser().getUserId() %>)</td>
<td><%=replyUser.getReply().getReplyContent() %></td>
<td><%=replyUser.getReply().getReplyDate() %></td>
<td><%=replyUser.getReply().getReplyCommentNo() %></td>
</tr>
<% } %>
</table>
</body>
</html>
13) ๐commentReplySelect1.jsp - ๐MyComment1 + ๐คMyReply ์ถ๋ ฅ
<%@page import="xyz.itwill.dto.MyReply"%>
<%@page import="java.util.List"%>
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@page import="xyz.itwill.dto.MyComment1"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
if(request.getParameter("commentNo")==null){
response.sendRedirect("commentUserListSelect2.jsp");
return;
}
//์ ๋ฌ๊ฐ(๊ฒ์๊ธ๋ฒํธ)์ ๋ฐํ๋ฐ์ ์ ์ฅ
int commentNo = Integer.parseInt(request.getParameter("commentNo"));
//์ ๋ฌ๋ฐ์ ๊ฒ์๊ธ๋ฒํธ์ ๋ํ ๊ฒ์๊ธ(1๊ฐ)์ ๊ฒ์ํ์ฌ ๋ฐํํ๋ DAOํด๋์ค์ ๋ฉ์๋ ํธ์ถ
MyComment1 comment = MyCommentDAO.getDAO().selectComment(commentNo);
//์ ๋ฌ๋ฐ์ ๊ฒ์๊ธ๋ฒํธ์ ๋ํ ๋๊ธ(0๊ฐ ์ด์)์ ๊ฒ์ํ์ฌ ๋ฐํํ๋ DAOํด๋์ค์ ๋ฉ์๋ ํธ์ถ
List<MyReply> replyList = MyCommentDAO.getDAO().selectCommentNoReplyList(commentNo);
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table{
border: 1px solid black;
border-collapse: collapse;
}
td{
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no{width: 100px;}
.name{width: 150px;}
.content{width: 250px;}
.date{width: 200px;}
.comment{width: 100px;}
</style>
</head>
<body>
<h1>๊ฒ์๊ธ๊ณผ ๋๊ธ๋ชฉ๋ก</h1>
<hr />
<table>
<tr>
<td width="200">๊ฒ์๊ธ๋ฒํธ</td>
<td width="200"><%=comment.getCommentNo() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ์์ฑ์</td>
<td width="200"><%=comment.getCommentId() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ๋ด์ฉ</td>
<td width="200"><%=comment.getCommentContent() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ์์ฑ์ผ</td>
<td width="200"><%=comment.getCommentDate() %></td>
</tr>
</table>
<br />
//<%--๋๊ธ๋ชฉ๋ก ์ถ๋ ฅ --%>
<table>
<tr>
<td class="no">๋๊ธ๋ฒํธ</td>
<td class="name">๋๊ธ์์ฑ์</td>
<td class="content">๋๊ธ๋ด์ฉ</td>
<td class="date">๋๊ธ์์ฑ์ผ</td>
<td class="comment">๊ฒ์๊ธ๋ฒํธ</td>
</tr>
<%if(replyList.isEmpty()) {%>
<tr>
<td colspan="5">๋๊ธ์ด ์กด์ฌํ์ง ์์ต๋๋ค.</td>
</tr>
<%} else {%>
<tr>
<%for(MyReply reply:replyList){ %>
<tr>
<td><%=reply.getReplyNo() %></td>
<td><%=reply.getReplyId() %></td>
<td><%=reply.getReplyContent() %></td>
<td><%=reply.getReplyDate() %></td>
<td><%=reply.getReplyCommentNo() %></td>
</tr>
<%} %>
</tr>
<%} %>
</table>
</body>
</html>
14) ๐commentReplySelect2.jsp - ๐คMyCommentReply ์ถ๋ ฅ
2๊ฐ ์กฐ์ธํ ๊ฐ์ฒด
<%@page import="xyz.itwill.dto.MyCommentReply"%>
<%@page import="xyz.itwill.dto.MyReply"%>
<%@page import="java.util.List"%>
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@page import="xyz.itwill.dto.MyComment1"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
if(request.getParameter("commentNo")==null){
response.sendRedirect("commentUserListSelect2.jsp");
return;
}
//์ ๋ฌ๊ฐ(๊ฒ์๊ธ๋ฒํธ)์ ๋ฐํ๋ฐ์ ์ ์ฅ
int commentNo = Integer.parseInt(request.getParameter("commentNo"));
//์ ๋ฌ๋ฐ์ ๊ฒ์๊ธ๋ฒํธ์ ๋ํ ๊ฒ์๊ธ(1๊ฐ)๊ณผ ๋๊ธ๋ชฉ๋ก(0๊ฐ ์ด์)์ ๊ฒ์ํ์ฌ ๋ฐํํ๋ DAOํด๋์ค์ ๋ฉ์๋ ํธ์ถ
MyCommentReply CommentReply = MyCommentDAO.getDAO().selectCommentReply(commentNo);
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table{
border: 1px solid black;
border-collapse: collapse;
}
td{
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no{width: 100px;}
.name{width: 150px;}
.content{width: 250px;}
.date{width: 200px;}
.comment{width: 100px;}
</style>
</head>
<body>
<h1>๊ฒ์๊ธ๊ณผ ๋๊ธ๋ชฉ๋ก</h1>
<hr />
<table>
<tr>
<td width="200">๊ฒ์๊ธ๋ฒํธ</td>
<td width="200"><%=CommentReply.getComment().getCommentNo() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ์์ฑ์</td>
<td width="200"><%=CommentReply.getComment().getCommentId() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ๋ด์ฉ</td>
<td width="200"><%=CommentReply.getComment().getCommentContent() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ์์ฑ์ผ</td>
<td width="200"><%=CommentReply.getComment().getCommentDate() %></td>
</tr>
</table>
<br />
<!--๋๊ธ๋ชฉ๋ก ์ถ๋ ฅ -->
<table>
<tr>
<td class="no">๋๊ธ๋ฒํธ</td>
<td class="name">๋๊ธ์์ฑ์</td>
<td class="content">๋๊ธ๋ด์ฉ</td>
<td class="date">๋๊ธ์์ฑ์ผ</td>
<td class="comment">๊ฒ์๊ธ๋ฒํธ</td>
</tr>
<%if(CommentReply.getReplyList().isEmpty()) {%>
<tr>
<td colspan="5">๋๊ธ์ด ์กด์ฌํ์ง ์์ต๋๋ค.</td>
</tr>
<%} else {%>
<tr>
<%for(MyReply reply:CommentReply.getReplyList()){ %>
<tr>
<td><%=reply.getReplyNo() %></td>
<td><%=reply.getReplyId() %></td>
<td><%=reply.getReplyContent() %></td>
<td><%=reply.getReplyDate() %></td>
<td><%=reply.getReplyCommentNo() %></td>
</tr>
<%} %>
</tr>
<%} %>
</table>
</body>
</html>
15) ๐commentReplyUserSelect.jsp - ๐คcommentReplyUser์ถ๋ ฅ
3๊ฐ ์กฐ์ธํ ๊ฐ์ฒด
<%@page import="xyz.itwill.dto.MyReplyUser"%>
<%@page import="xyz.itwill.dao.MyCommentDAO"%>
<%@page import="xyz.itwill.dto.MyCommentReplyUser"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
if(request.getParameter("commentNo")==null){
response.sendRedirect("commentUserListSelect2.jsp");
return;
}
//์ ๋ฌ๊ฐ(๊ฒ์๊ธ๋ฒํธ)์ ๋ฐํ๋ฐ์ ์ ์ฅ
int commentNo = Integer.parseInt(request.getParameter("commentNo"));
//์ ๋ฌ๋ฐ์ ๊ฒ์๊ธ๋ฒํธ์ ๋ํ ๊ฒ์๊ธ(1๊ฐ)๊ณผ ๋๊ธ๋ชฉ๋ก(0๊ฐ ์ด์)์ ๊ฒ์ํ์ฌ ๋ฐํํ๋ DAOํด๋์ค์ ๋ฉ์๋ ํธ์ถ
MyCommentReplyUser commentReplyUser = MyCommentDAO.getDAO().selectCommentReplyUser(commentNo);
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MYBATIS</title>
<style type="text/css">
table{
border: 1px solid black;
border-collapse: collapse;
}
td{
border: 1px solid black;
text-align: center;
padding: 3px;
}
.no{width: 100px;}
.name{width: 150px;}
.content{width: 250px;}
.date{width: 200px;}
.comment{width: 100px;}
</style>
</head>
<body>
<h1>๊ฒ์๊ธ๊ณผ ๋๊ธ๋ชฉ๋ก</h1>
<hr />
<table>
<tr>
<td width="200">๊ฒ์๊ธ๋ฒํธ</td>
<td width="200"><%=commentReplyUser.getCommentNo() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ์์ฑ์</td>
<td width="200"><%=commentReplyUser.getUser().getUserName() %>(<%=commentReplyUser.getUser().getUserId() %>)</td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ๋ด์ฉ</td>
<td width="200"><%=commentReplyUser.getCommentContent() %></td>
</tr>
<tr>
<td width="200">๊ฒ์๊ธ ์์ฑ์ผ</td>
<td width="200"><%=commentReplyUser.getCommentDate() %></td>
</tr>
</table>
<br />
<!--๋๊ธ๋ชฉ๋ก ์ถ๋ ฅ -->
<table>
<tr>
<td class="no">๋๊ธ๋ฒํธ</td>
<td class="name">๋๊ธ์์ฑ์</td>
<td class="content">๋๊ธ๋ด์ฉ</td>
<td class="date">๋๊ธ์์ฑ์ผ</td>
<td class="comment">๊ฒ์๊ธ๋ฒํธ</td>
</tr>
<%if(commentReplyUser.getReplyUserList()==null ||commentReplyUser.getReplyUserList().isEmpty()) {%>
<tr>
<td colspan="5">๋๊ธ์ด ์กด์ฌํ์ง ์์ต๋๋ค.</td>
</tr>
<%} else {%>
<tr>
<%for(MyReplyUser replyUser:commentReplyUser.getReplyUserList()){ %>
<tr>
<td><%=replyUser.getReply().getReplyNo() %></td>
<td><%=replyUser.getUser().getUserId() %>(<%=replyUser.getUser().getUserName() %>)</td>
<td><%=replyUser.getReply().getReplyContent() %></td>
<td><%=replyUser.getReply().getReplyDate() %></td>
<td><%=replyUser.getReply().getReplyCommentNo() %></td>
</tr>
<%} %>
</tr>
<%} %>
</table>
</body>
</html>